Get the Dev Stack That Does the Heavy Lifting
Claim Your FREE Copy
Dev Stack Starter Guide
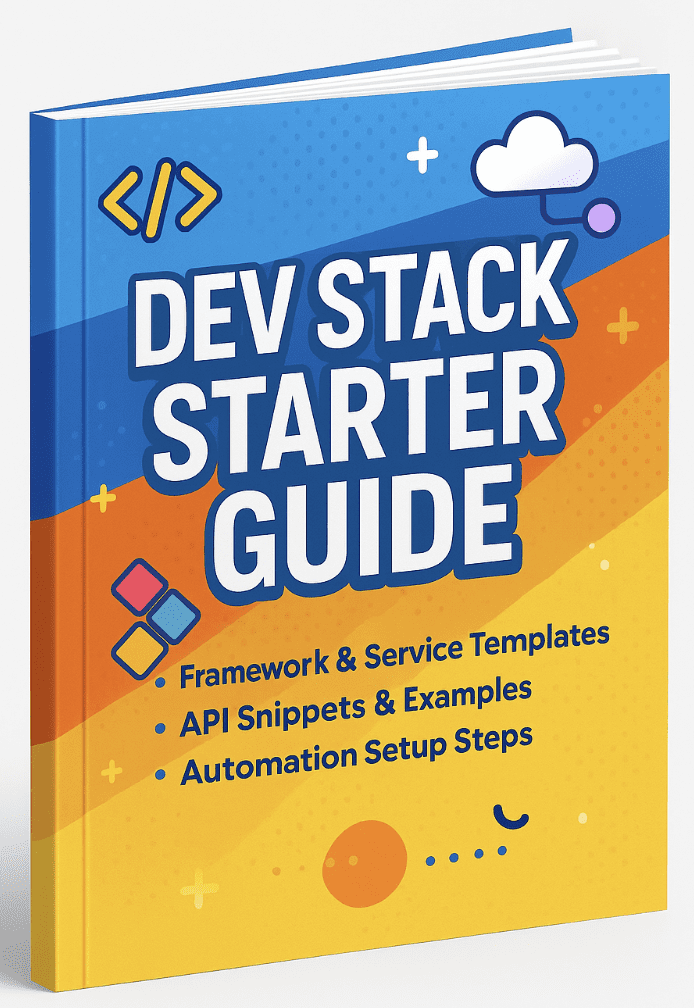
Discover the automation tools top developers are turning to for faster launches and better systems.
- ✅ Pre-built API Scripts & Examples
- ✅ Proven Workflow Blueprints
- ✅ Hands-On CRM + Funnel Setup
- ✅ Designed for Developers, Freelancers & SaaS Creators
Recent Posts